Vue 3 Global Filters
May 12, 2021
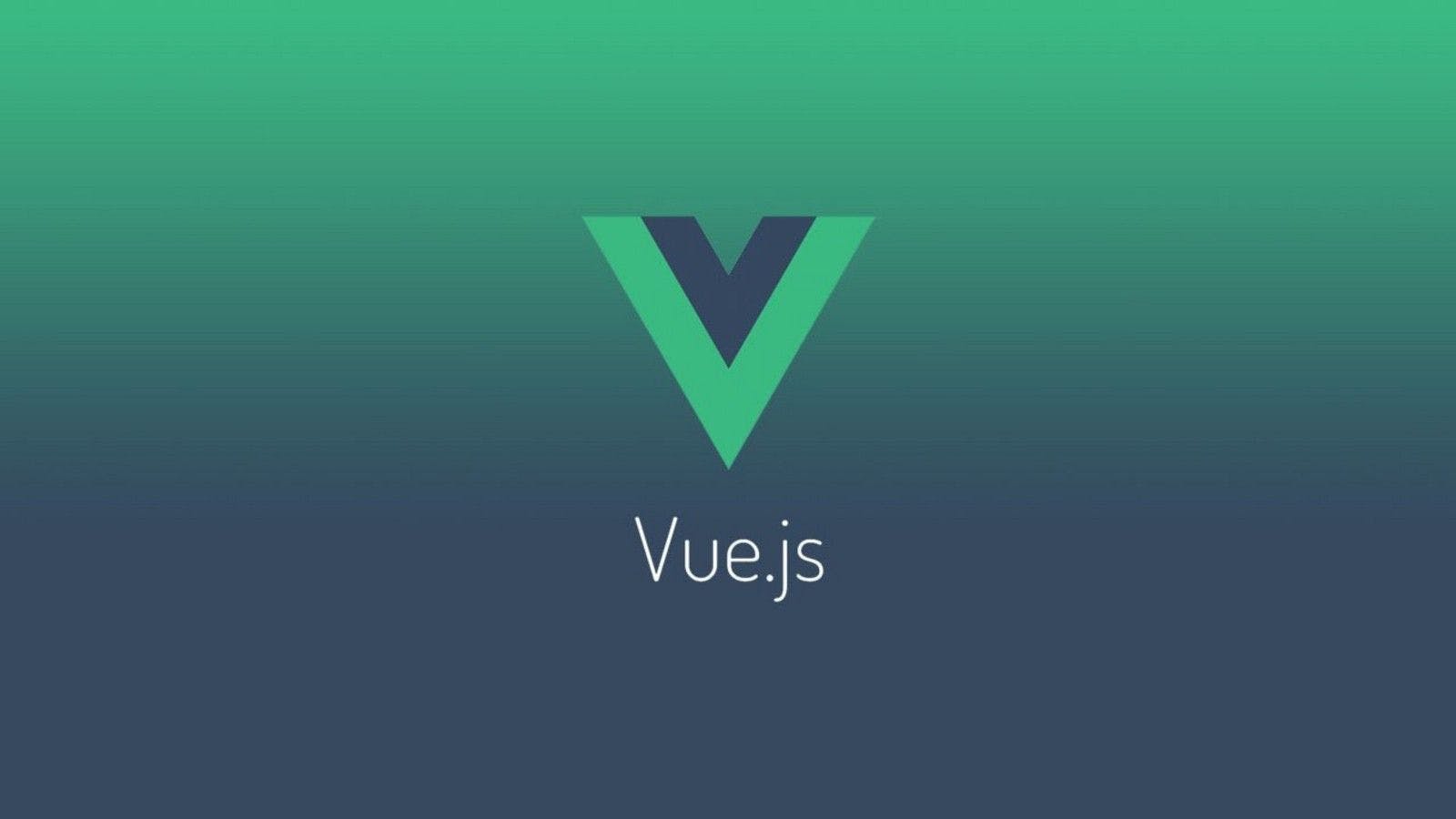
As of Vue 3, Global Filters are no longer supported. 🙀
Our team uses these frequently in our projects for things like currency
and prettyDate
formatting. In Vue 2, these were implemented by adding the following in your app.js
or root js file:
Vue.filter('niceTimestamp', (val) => { return new Date(val).toLocaleString() })
The recommendation for Global Filters from the Vue 3 documentation is as follows:
If you are using filters that were globally registered and then used throughout your app, it's likely not convenient to replace them with computed properties or methods in each individual component.
Instead, you can make your global filters available to all components through globalProperties:
This would look like:
// app.js const app = createApp(App) app.config.globalProperties.$filters = { currencyUSD(value) { return '$' + value } }
And then in any of your components, you can access them via the $filters
object.
$filters.currencyUSD(accountBalance)
🔥 Tip for implementing this on Laravel + Inertia projects
The default scaffolding for the app.js
file will include the following:
createApp({ render: () => h(InertiaApp, { initialPage: JSON.parse(el.dataset.page), resolveComponent: (name) => require(./Pages/${name}).default, }), }) .mixin({ methods: { route } }) .use(InertiaPlugin) .mount(el);
So, when you follow the documentation, your first inclination will be to assign the entire block above to const app
or similar. However, this will not work.
It will not work because we need to assign createApp()
(which returns the Root Component) only to the const app
. Then we can add the globalFilters
, etc. A working example is below:
// assign the Root Component const app = createApp({ render: () => h(InertiaApp, { initialPage: JSON.parse(el.dataset.page), resolveComponent: (name) => require(./Pages/${name}).default, }), }) // initialize with options app.mixin({ methods: { route } }) .use(InertiaPlugin) .mount(el); // apply global filters app.config.globalProperties.$filters = { currency(value) { return '$' + new Intl.NumberFormat('en-US').format(value) } }