Salesforce :: Recursively Fetching All Records
April 15, 2021
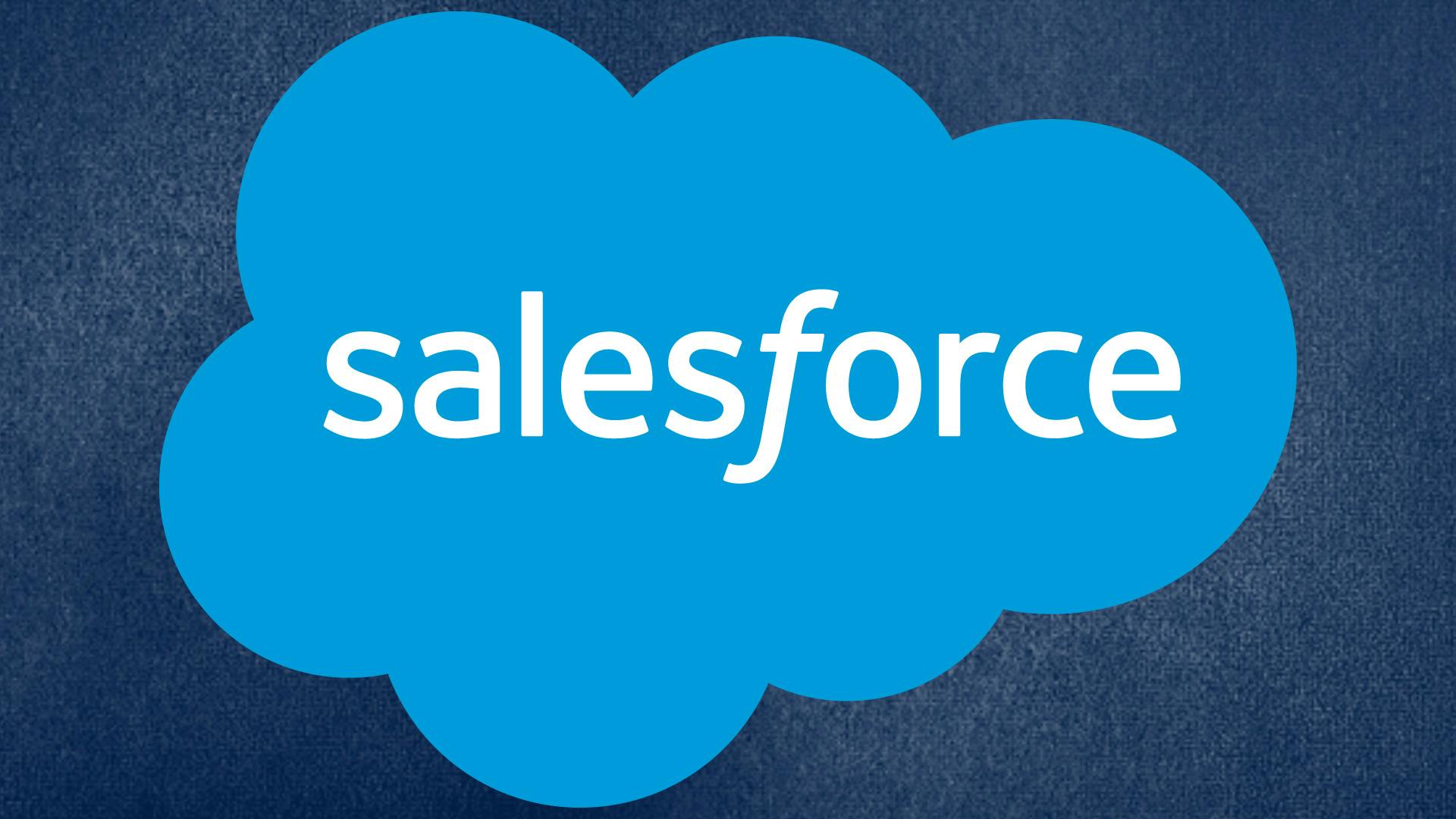
In my previous post, we discussed an straight forward approach to connecting to the Salesforce REST API.
While working on this app for our client, one of the requirements was to display a list of Inventory for printing / exporting. This is a situation when the built in Pagination from the Salesforce API is not your friend.
By default it returns 200 records, but according to the documentation you can pass a header to retrieve up to 2000 at a time. This is what we needed as some of the lists to export were 4000+ items.
We attempted the header approach but were always greeted with a max result of 200. 😏
Since the Salesforce API Query Endpoint does return a nextRecordsUrl
property if there are more results, we just needed a way to keeping querying until there were not "more records" to fetch.
We were able to accomplish this by creating a fetchRecords()
method initiates the first query, and if the result returns a nextRecordsUrl
, the we call the same method, but this time the $query
parameter is populated with the nextRecordsUrl
.
Finally, we return a fully populated $inventory
object.
private function fetchRecords(Client $client, string $accessToken, string $query, array &$inventory) { $response = $client->get($query, [ RequestOptions::HEADERS => [ 'Authorization' => 'Bearer ' . $accessToken, 'X-PrettyPrint' => 1, ], ]);1 $content = json_decode($response->getBody());23 foreach ($content->records as $record) {4 $inventory[] = $record;5 }67 if (isset($content->nextRecordsUrl)) {8 return $this->fetchRecords($client, $accessToken, $content->nextRecordsUrl, $inventory);9 }1011 return collect($inventory);12}
There is nothing earth shattering about the logic above, but our searches on how to accomplish fetching all the records did not lead to any other solutions. Hopefully this will help someone that is facing the same problem. ✌🏼